Recipe api
Javascript
Timeline:
1 weeks Duration
Role:
Developer
Tools:
HTML5 CSS3 jQuery JavaScript Bootstrap
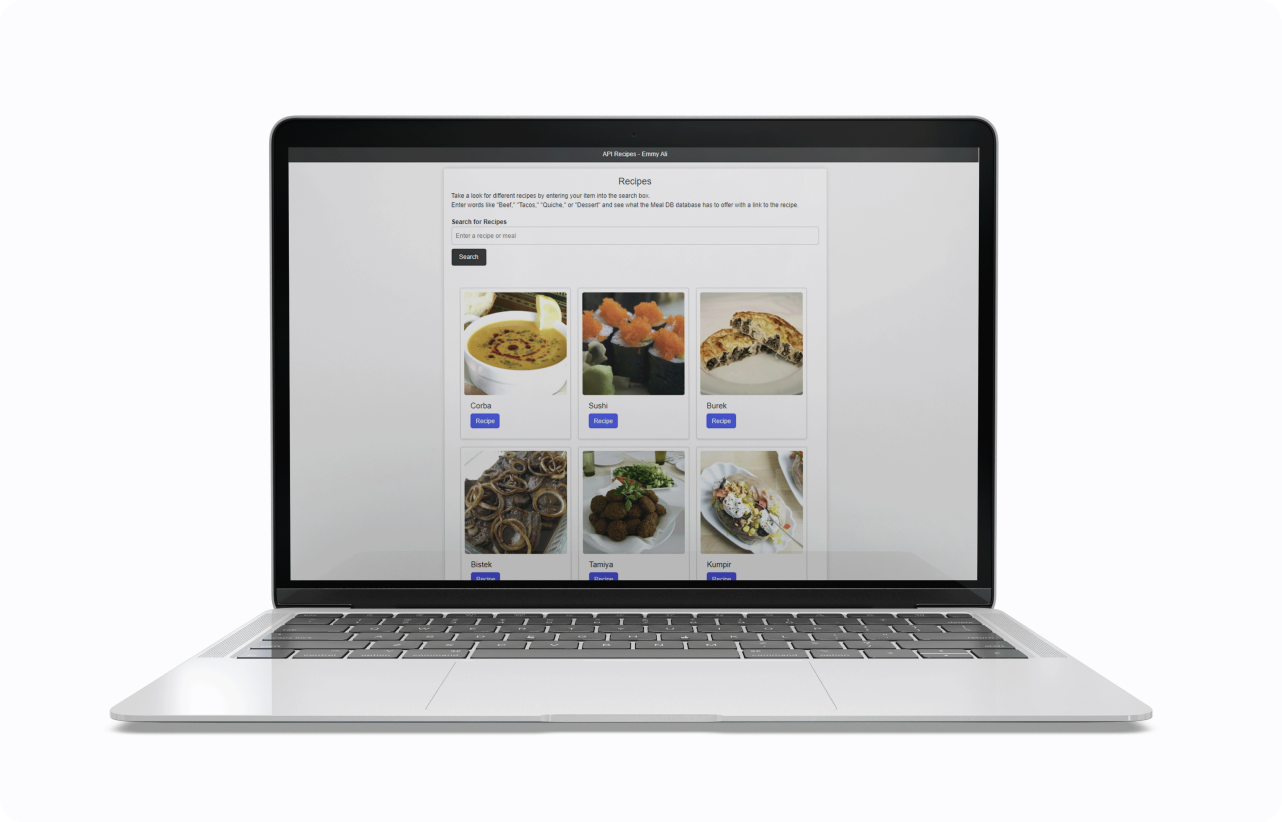
Overview:
I developed a web tool driven by my passion for cooking. This tool, I have created using APIs, jQuery, and Bootstrap, and enables users to effortlessly discover and explore various recipes. Leveraging 'The Meal DB' API, users can dynamically retrieve recipe details, making it a quick and user-friendly way to find the perfect meal inspiration.
01/08
The Process:
I started this project by creating a mockup in Figma of how I wanted the application to look. Having the layout mapped out allowed for faster coding since the design was complete. I then moved on to coding the HTML markup according to the mockup.
In JavaScript section, I created a global variable `searchItem` and `loadRecipes()` function, which clears the previous search results and adds a spinner while fetching the data. From the other hand `clearRecipes()` function clears the search result container.
function addToContainer(result) {
var myContainer = document.querySelector('#recipe-rows-container');
if (result.meals !== null && result.meals !== '' && result.meals !== undefined) {
// Loop through the array of objects
for (var i = 0; i < result.meals.length; i++) {
var card = document.createElement('div');
card.classList.add('card');
// Add Images and append them to the card
var img = document.createElement('img');
img.classList.add('card-img-top');
img.src = result.meals[i].strMealThumb;
img.alt = result.meals[i].strMeal + ' recipe';
var cardBody = document.createElement('div');
cardBody.classList.add('card-body');
// Create the title for each recipe name in the card
var title = document.createElement('h5');
title.classList.add('card-title');
title.innerText = result.meals[i].strMeal;
// Create the "Recipe" button
var recipeButton = document.createElement('button');
recipeButton.classList.add('btn', 'btn-primary');
recipeButton.innerText = 'Recipe';
// Use the meal ID as a unique identifier for the recipe
recipeButton.setAttribute('data-meal-id', result.meals[i].idMeal);
recipeButton.addEventListener('click', function() {
var mealId = this.getAttribute('data-meal-id');
showRecipeDetails(mealId);
});
// Append elements to the card
cardBody.appendChild(title);
cardBody.appendChild(recipeButton);
card.appendChild(img);
card.appendChild(cardBody);
// Append the card to the recipe-rows-container
myContainer.appendChild(card);
}
}
}
I have created recipe card with loops through the API response. Each card includes and image, recipe title and a “Recipe” button. To add functionality for recipe details, I have added a `showRecipeDetails(recipeId)` function, which fetches detailed recipe information using another API call. This function displays the details in an alert, including title, category, area, and instructions.
I have also included external libraries, like jQuery for AJAX functionality and Bootstrap JavaScript for enhanced UI components.
function showRecipeDetails(recipeId) {
// Fetching the recipe details using another AJAX call to the API
$.ajax('https://www.themealdb.com/api/json/v1/1/lookup.php?i=' + recipeId).done(function(recipeDetails) {
// Handle the response and display the details
if (recipeDetails.meals && recipeDetails.meals.length > 0) {
var selectedRecipe = recipeDetails.meals[0];
// Extract the recipe title, instructions, category, and area
var recipeTitle = selectedRecipe.strMeal;
var recipeInstructions = selectedRecipe.strInstructions;
var recipeCategory = selectedRecipe.strCategory;
var recipeArea = selectedRecipe.strArea;
// Display the details in a modal or a separate section
displayRecipeDetails(recipeTitle, recipeInstructions, recipeCategory, recipeArea);
} else {
alert('Recipe not found');
}
})
.fail(function(xhr, status, error) {
console.log("Error: " + error);
});
}
02/08
Final Takeaways:
Building this recipe search project was fun as it aligned with my passion for cooking and allowed me to apply web development skills. Using "The Meal DB" API demonstrated my ability to work with external data, and the interactive features made the development process enjoyable. As the project progresses, thorough testing will be done to ensure a smooth user experience.
Solving challenges, achieving practical results, and aligning the project with my interests contributed to a sense of accomplishment and fulfillment.
03/08
Final Design:
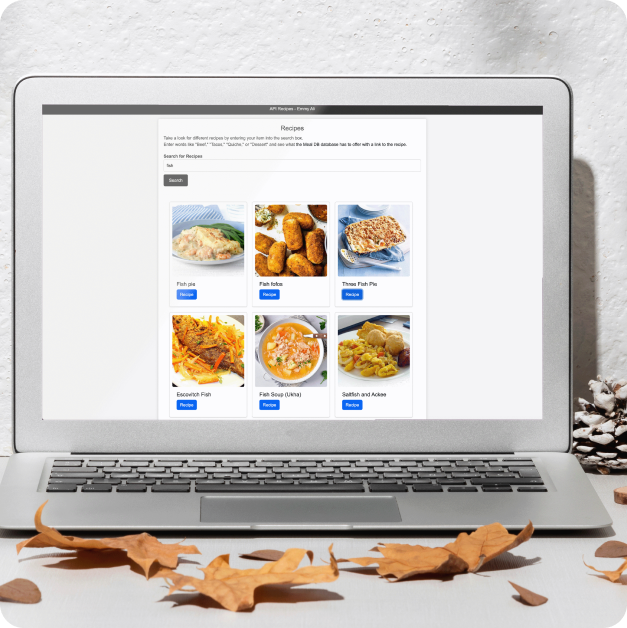
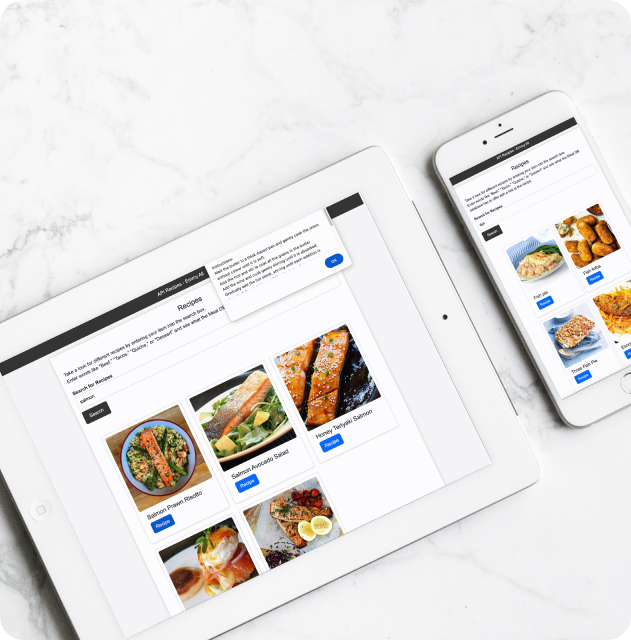
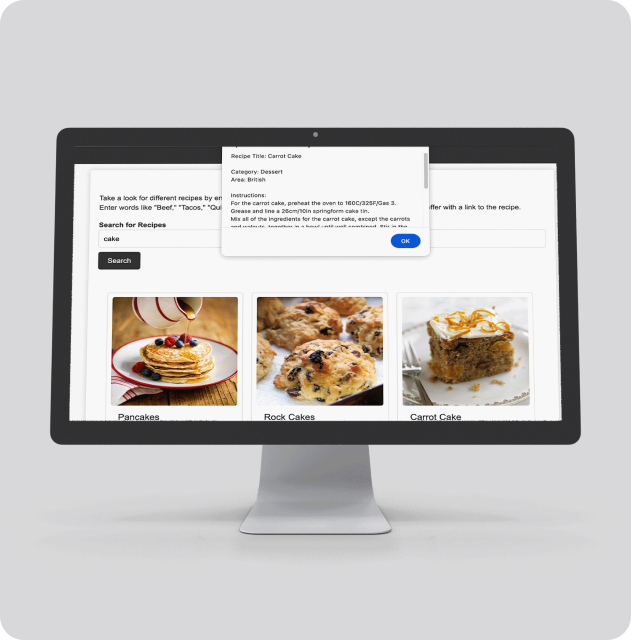
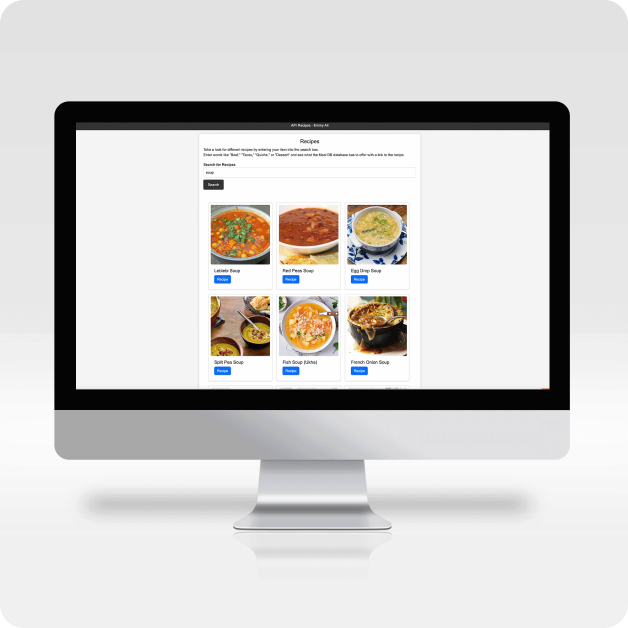